Arduino Programming
Arduino because they think programming is scary. Because of this, we wanted to make sure this tutorial was written for the absolute beginner with no experience whatsoever. This tutorial is a high level view of all the parts and pieces of the Arduino ecosystem. In future posts, we will take you step by step in creating your first simple. C and Arduino programming (+best practices) Become more autonomous when programming on Arduino, and rely less on copying/pasting Write code and practice instead of just sitting and watching Build a very basic Arduino circuit.
- Arduino Tutorial
- Arduino Function Libraries
- Arduino Advanced
- Arduino Projects
- Arduino Sensors
- Motor Control
- Arduino And Sound
- Arduino Useful Resources
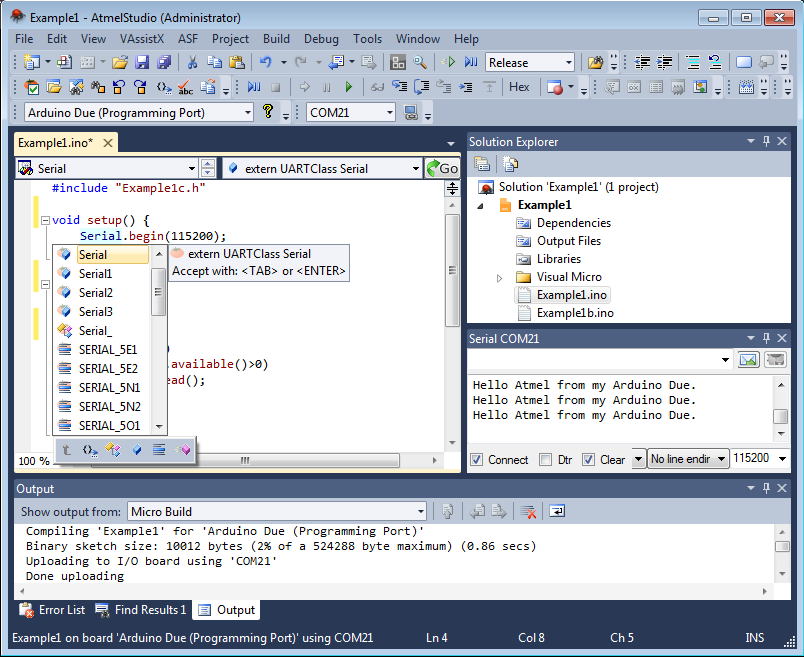
- Selected Reading
This example demonstrates the use of the analogWrite() function in fading an LED off. AnalogWrite uses pulse width modulation (PWM), turning a digital pin on and off very quickly with different ratios between on and off, to create a fading effect.
Arduino Programming C++
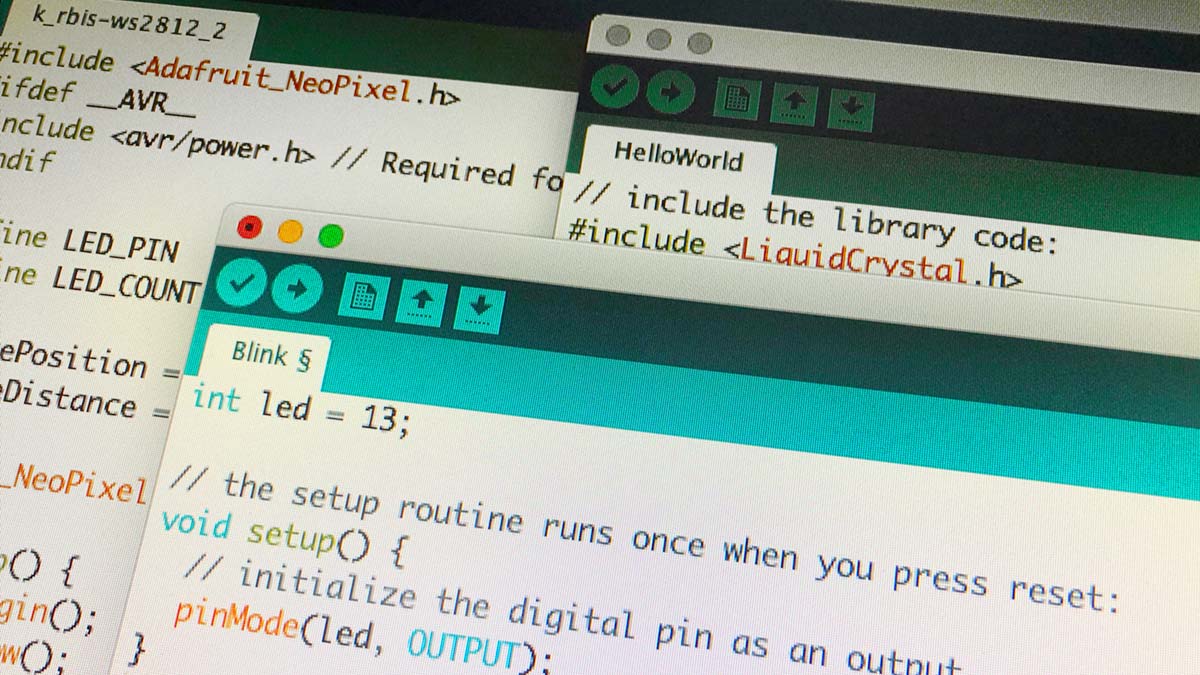
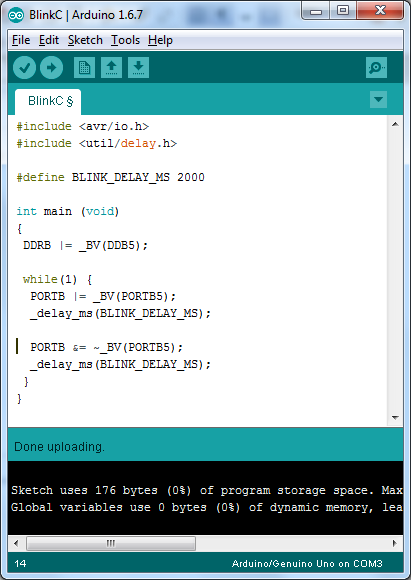

Components Required
You will need the following components −
- 1 × Breadboard
- 1 × Arduino Uno R3
- 1 × LED
- 1 × 330Ω Resistor
- 2 × Jumper
Procedure
Follow the circuit diagram and hook up the components on the breadboard as shown in the image given below.
Note − To find out the polarity of an LED, look at it closely. The shorter of the two legs, towards the flat edge of the bulb indicates the negative terminal.
Components like resistors need to have their terminals bent into 90° angles in order to fit the breadboard sockets properly. You can also cut the terminals shorter.
Sketch
Open the Arduino IDE software on your computer. Coding in the Arduino language will control your circuit. Open the new sketch File by clicking New.
Arduino Code
Code to Note
After declaring pin 9 as your LED pin, there is nothing to do in the setup() function of your code. The analogWrite() function that you will be using in the main loop of your code requires two arguments: One, telling the function which pin to write to and the other indicating what PWM value to write.
In order to fade the LED off and on, gradually increase the PWM values from 0 (all the way off) to 255 (all the way on), and then back to 0, to complete the cycle. In the sketch given above, the PWM value is set using a variable called brightness. Each time through the loop, it increases by the value of the variable fadeAmount.
If brightness is at either extreme of its value (either 0 or 255), then fadeAmount is changed to its negative. In other words, if fadeAmount is 5, then it is set to -5. If it is -5, then it is set to 5. The next time through the loop, this change causes brightness to change direction as well.
analogWrite() can change the PWM value very fast, so the delay at the end of the sketch controls the speed of the fade. Try changing the value of the delay and see how it changes the fading effect.
Arduino Programming Tutorial
Result
Arduino Programming Course
You should see your LED brightness change gradually.
Topic | Replies | Views | Activity |
---|---|---|---|
About the Programming Questions category | 0 | 142 | April 12, 2021 |
How to get the best out of this forum | 0 | 5368 | November 4, 2020 |
Useful links - check here for reference posts / tutorials | 1 | 95765 | April 18, 2021 |
Read this before posting a programming question ... | 30 | 384237 | April 18, 2021 |
How to resolve 'scale' not declared | 6 | 10 | April 25, 2021 |
I2C. Error when I try to send the roll angle between two Arduinos | 3 | 16 | April 25, 2021 |
PM2.5 Sensor Project: Possible Library Conflict | 3 | 17 | April 25, 2021 |
Quaternion Does Not Name A Type | 5 | 8 | April 25, 2021 |
Stuck on fahrenheit | 0 | 6 | April 25, 2021 |
I need BIG help with this project of mine! MK II | 20 | 138 | April 25, 2021 |
3 out of 4 timer pins don't do anything | 2 | 20 | April 25, 2021 |
Arduino | raspberry pi - communication | 1 | 13 | April 24, 2021 |
Help with CapSense Piano! | 20 | 28 | April 24, 2021 |
Program size difference with different enum types | 16 | 55 | April 24, 2021 |
Make servo move by serial commands | 8 | 20 | April 24, 2021 |
Issues creating a library with char arrays | 18 | 29 | April 24, 2021 |
RBG strip arduino | 3 | 13 | April 24, 2021 |
How do I write this IF statement? | 22 | 144 | April 24, 2021 |
Connections solar cell + LCD display without the USB power supply of the Arduino UNO | 1 | 12 | April 24, 2021 |
Analog signal to enable DC motor for set time | 5 | 23 | April 24, 2021 |
Detect hardware before setup, or, recreating clients in run time | 15 | 28 | April 24, 2021 |
When button is pressed in loop question | 11 | 36 | April 24, 2021 |
Class and constructor. | 27 | 498 | April 24, 2021 |
Problem lighting lights up | 61 | 181 | April 24, 2021 |
Interrupt like environment within normal code | 6 | 64 | April 24, 2021 |
Serial connection dont display anything on bare bone arduino | 9 | 21 | April 24, 2021 |
Arduino based hand sanitizer | 10 | 34 | April 24, 2021 |
Two sensor values only if there is a change | 3 | 14 | April 24, 2021 |
(.text+0x0): multiple definition of ... running out of ideas | 15 | 129 | April 24, 2021 |
Problem with 'i' was not declared in this scope | 6 | 30 | April 24, 2021 |
